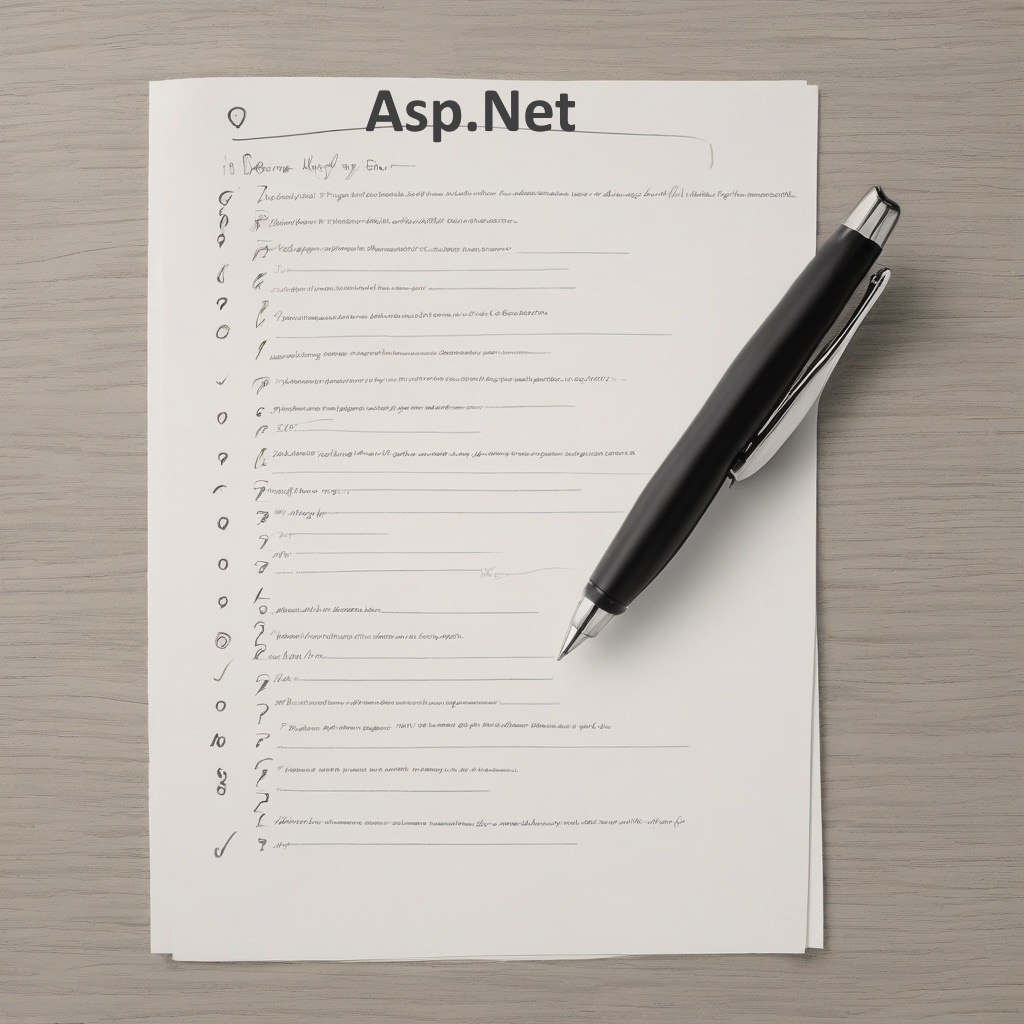
Starting a career in ASP.NET development can be an exciting journey, but it often begins with facing interviews that can feel daunting. To help you prepare effectively, here are the top 20 ASP.NET interview questions along with detailed answers that will not only boost your confidence but also showcase your knowledge and skills.
1. What is ASP.NET?
ASP.NET is a web development framework developed by Microsoft. It allows developers to build dynamic web applications and services using languages like C# or Visual Basic.
2. Differentiate between ASP.NET Web Forms and ASP.NET MVC.
ASP.NET Web Forms follows a traditional event-driven programming model, while ASP.NET MVC (Model-View-Controller) separates the application into three main components: the model (data), the view (UI), and the controller (logic).
3. Explain the ASP.NET Page Life Cycle.
The ASP.NET Page Life Cycle defines the stages a web page goes through from initialization to disposal. It includes stages like initialization, load, postback data handling, rendering, and unloading.
4. What is ViewState in ASP.NET?
ViewState is used to persist the state of server-side objects across postbacks. It’s stored in a hidden field on the page and helps in maintaining the state of controls like TextBoxes, DropDownLists, etc., between postbacks.
5. How does ASP.NET handle session state?
ASP.NET supports various modes for session state management, including InProc (in-process), StateServer, SQLServer, and Custom. These modes define where session data is stored and how it is managed across requests.
6. What are the different types of caching in ASP.NET?
ASP.NET supports several types of caching, including Output Caching (caching the entire page), Data Caching (caching data objects), and Application Caching (caching application-wide data).
7. Explain the role of Global.asax in ASP.NET.
Global.asax is a file where you can write application-level events and code. It includes events like Application_Start (executed when the application starts) and Application_End (executed when the application shuts down).
8. How does ASP.NET handle authentication and authorization?
ASP.NET provides built-in authentication mechanisms like Forms authentication, Windows authentication, and Passport authentication. Authorization is managed through roles and permissions assigned to users.
9. What is the difference between ASP.NET Web API and ASP.NET MVC?
ASP.NET Web API is designed for building RESTful APIs, while ASP.NET MVC is focused on building web applications following the MVC pattern.
10. What are Action Filters in ASP.NET MVC?
Action Filters are attributes that can be applied to controller actions to perform logic before or after the action is executed. Examples include Authorization filters and Action filters.
11. Explain the concept of Dependency Injection (DI) in ASP.NET.
Dependency Injection is a design pattern where dependencies of a class are injected from the outside rather than created within the class. It promotes loose coupling and easier testing.
12. What is Entity Framework in ASP.NET?
Entity Framework is an Object-Relational Mapping (ORM) framework provided by Microsoft. It simplifies database operations by allowing developers to work with database entities as objects in their code.
13. How do you handle exceptions in ASP.NET?
Exceptions in ASP.NET can be handled using try-catch blocks. Additionally, you can use global error handling in the Global.asax file to catch unhandled exceptions.
14. What is Razor syntax in ASP.NET?
Razor is a syntax used in ASP.NET MVC for embedding server-side code (C# or VB.NET) into views. It provides a clean and concise way to write dynamic content in HTML.
15. Explain the concept of Bundling and Minification in ASP.NET.
Bundling and Minification are techniques used to improve website performance by combining and compressing CSS and JavaScript files, reducing the number of HTTP requests.
16. What is the use of the App_Code folder in ASP.NET?
The App_Code folder is used to store code files (classes, business logic, utility methods) that are automatically compiled and made available to the application.
17. How does ASP.NET handle State Management?
ASP.NET supports various state management techniques like ViewState, Session state, Cookies, Application state, and QueryStrings to maintain state across multiple requests.
18. Explain the concept of Partial Views in ASP.NET MVC.
Partial Views are reusable components in ASP.NET MVC that represent a part of a view. They can be rendered within other views, allowing for modular and maintainable UI development.
19. What are Web API Controllers in ASP.NET?
Web API Controllers in ASP.NET are specialized controllers used for building RESTful APIs. They handle HTTP requests and return data in JSON or XML format.
20. How can you improve the performance of an ASP.NET application?
Performance optimization techniques include using caching, minimizing database calls, bundling and minifying static resources, optimizing code, using asynchronous programming, and implementing proper indexing in databases.
These interview questions cover a wide range of ASP.NET concepts and are crucial for freshers looking to kickstart their careers in ASP.NET development. Practising these questions and understanding the underlying concepts will undoubtedly help you ace your ASP.NET interviews with confidence. Good luck!