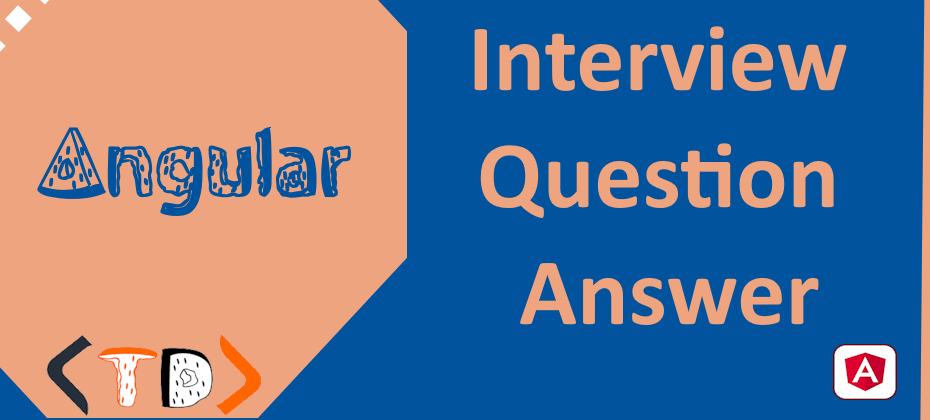
Here are some of the best questions and answers for Angular technology interviews, covering a range of topics from basic to advanced:
Basic Questions
- What is Angular and why is it used?
- Angular is a platform and framework for building single-page client applications using HTML and TypeScript. It’s used for its powerful features like two-way data binding, dependency injection, and a component-based architecture, which simplify the development and testing of web applications.
- What is the Angular CLI and what are its advantages?
- The Angular CLI (Command Line Interface) is a tool to initialize, develop, scaffold, and maintain Angular applications. Its advantages include simplifying the setup process, generating boilerplate code, performing various development tasks, and ensuring best practices and consistency across projects.
- What is a component in Angular?
- A component is the basic building block of an Angular application. It consists of a TypeScript class that defines the behavior and properties, an HTML template that defines the view, and CSS styles that define the appearance.
- What is data binding in Angular?
- Data binding is a mechanism to coordinate the data between the model and the view. Angular supports several types of data binding, including interpolation, property binding, event binding, and two-way binding.
Intermediate Questions
- Explain Angular services and dependency injection.
- Services in Angular are singleton objects that encapsulate reusable logic and can be shared across components. Dependency injection is a design pattern used to implement IoC (Inversion of Control), allowing Angular to inject dependencies (like services) into components, directives, and other services at runtime.
- What is RxJS and how is it used in Angular?
- RxJS (Reactive Extensions for JavaScript) is a library for reactive programming using observables. In Angular, RxJS is used for handling asynchronous operations, such as HTTP requests, user inputs, and other events. Observables and operators in RxJS allow for powerful and flexible asynchronous data handling.
- What are Angular directives and their types?
- Directives are classes that add behavior to elements in Angular applications. There are three types of directives:
- Components: Directives with a template.
- Structural directives: Modify the DOM layout by adding, removing, or manipulating elements (e.g.,
*ngIf
,*ngFor
). - Attribute directives: Change the appearance or behavior of an element (e.g.,
ngClass
,ngStyle
).
- Directives are classes that add behavior to elements in Angular applications. There are three types of directives:
Advanced Questions
- What is the Angular lifecycle and its hooks?
- Angular lifecycle refers to the sequence of events from the creation to the destruction of a component. Lifecycle hooks are methods that allow developers to tap into key moments in this lifecycle. Important hooks include
ngOnInit()
,ngOnChanges()
,ngDoCheck()
,ngAfterViewInit()
,ngAfterContentInit()
,ngOnDestroy()
, and others.
- Angular lifecycle refers to the sequence of events from the creation to the destruction of a component. Lifecycle hooks are methods that allow developers to tap into key moments in this lifecycle. Important hooks include
- Explain Angular’s change detection mechanism.
- Angular’s change detection mechanism updates the view whenever the model changes. It uses the
zone.js
library to detect changes in application state and trigger the necessary updates. Angular employs a tree of change detectors to check each component for changes and update the view accordingly. TheOnPush
change detection strategy can be used for performance optimization by checking changes only when input properties change.
- Angular’s change detection mechanism updates the view whenever the model changes. It uses the
- What is lazy loading and how is it implemented in Angular?
- Lazy loading is a design pattern to load modules only when they are required, which can significantly reduce the initial load time of an application. In Angular, lazy loading is implemented using the
loadChildren
property in the route configuration. This loads the specified module only when the associated route is accessed.
const routes: Routes = [ { path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) } ];
- Lazy loading is a design pattern to load modules only when they are required, which can significantly reduce the initial load time of an application. In Angular, lazy loading is implemented using the
- What are Angular pipes and how are they used?
- Pipes are a way to transform data in Angular templates. They take data as input and return the transformed data as output. Angular provides built-in pipes like
DatePipe
,UpperCasePipe
,LowerCasePipe
,CurrencyPipe
, etc. Custom pipes can also be created by implementing thePipeTransform
interface.
<p>{{ today | date:'shortDate' }}</p>
- Pipes are a way to transform data in Angular templates. They take data as input and return the transformed data as output. Angular provides built-in pipes like
- How does Angular handle form validation?
- Angular supports both template-driven and reactive forms for form validation. Validators can be built-in or custom. Template-driven forms use Angular directives to create and manage forms, while reactive forms use a more explicit and programmatic approach.
// Example of reactive form validation this.myForm = this.fb.group({ name: ['', [Validators.required, Validators.minLength(4)]], email: ['', [Validators.required, Validators.email]] });
Expert-Level Questions
- What is Ahead-of-Time (AOT) compilation and what are its benefits?
- AOT compilation converts Angular HTML and TypeScript code into efficient JavaScript code during the build process, before the browser downloads and runs the code. Benefits of AOT include faster rendering, fewer asynchronous requests, smaller Angular framework download size, and earlier detection of template errors.
- Explain the role of NgModules in Angular.
- NgModules are containers for a cohesive block of code dedicated to an application domain, a workflow, or a set of capabilities. They help organize an application into cohesive blocks of functionality and configure the injector and the compiler. Each Angular application has at least one NgModule, the root module, which is used to bootstrap the application.
- What are Angular elements and how are they useful?
- Angular elements allow Angular components to be used as custom elements (web components) outside of an Angular application. This makes it possible to integrate Angular components into other environments, such as static HTML pages or other frameworks.
import { createCustomElement } from '@angular/elements'; import { Injector } from '@angular/core'; const el = createCustomElement(MyComponent, { injector }); customElements.define('my-component', el);
These questions and answers cover a broad spectrum of Angular topics, from basic concepts to more advanced and expert-level functionalities. Being well-versed in these areas will help you confidently tackle Angular-related interview questions.