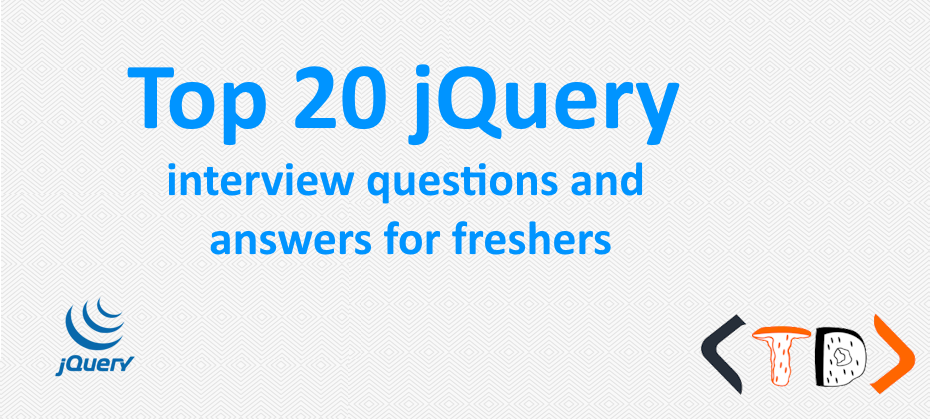
If you’re a fresher preparing for a jQuery-related job interview, it’s essential to be well-versed in both the basics and some advanced concepts of this powerful JavaScript library. To help you ace your interview, we have compiled a list of the top 20 jQuery interview questions and answers. These questions cover fundamental aspects of jQuery that are commonly asked in interviews.
1. What is jQuery?
Answer: jQuery is a fast, small, and feature-rich JavaScript library. It simplifies tasks like HTML document traversal and manipulation, event handling, and animation, making it easier to use JavaScript on your website.
2. Why is jQuery used?
Answer: jQuery is used to simplify complex JavaScript code, making it easier to manipulate the DOM, handle events, create animations, and perform AJAX requests.
3. What are the features of jQuery?
Answer: Key features of jQuery include DOM manipulation, event handling, CSS manipulation, effects and animations, AJAX support, and extensibility via plugins.
4. How do you include jQuery in a project?
Answer: You can include jQuery by linking to a CDN (Content Delivery Network) or downloading the jQuery library and linking it locally. Example using CDN:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
5. Explain the jQuery selector and give an example.
Answer: jQuery selectors are used to select and manipulate HTML elements. They are based on CSS selectors. Example:
$('p').css('color', 'blue'); // Selects all <p> elements and changes their color to blue
6. What is the difference between $(document).ready() and $(window).on(‘load’)?
Answer: $(document).ready()
executes the function as soon as the DOM is fully loaded, while $(window).on('load')
waits until the entire page, including all assets like images, is fully loaded.
7. What are jQuery plugins?
Answer: jQuery plugins are reusable pieces of code that can be added to the jQuery library to extend its functionality. Developers can create their own plugins or use plugins created by others.
8. How do you create a jQuery plugin?
Answer: A basic jQuery plugin can be created by extending the jQuery.fn object. Example:
(function($) {
$.fn.myPlugin = function() {
// Plugin code here
return this; // Maintain chainability
};
}(jQuery));
9. What is the use of the each()
function in jQuery?
Answer: The each()
function is used to iterate over a set of matched elements and execute a function for each element. Example:
$('li').each(function(index) {
$(this).css('color', 'blue');
});
10. How do you handle events in jQuery?
Answer: jQuery provides methods like .on()
, .click()
, .hover()
, etc., to handle events. Example:
$('#myButton').on('click', function() {
alert('Button clicked!');
});
11. What is event delegation in jQuery?
Answer: Event delegation involves using a single event listener to manage all events of a particular type within a parent element. It uses event bubbling to handle events more efficiently. Example:
$('#parent').on('click', '.child', function() {
alert('Child clicked!');
});
12. Explain the ajax()
method in jQuery.
Answer: The ajax()
method is used to perform asynchronous HTTP requests. It provides options to configure the request. Example:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
console.log(response);
},
error: function(error) {
console.error(error);
}
});
13. What is chaining in jQuery?
Answer: Chaining allows you to execute multiple jQuery methods on the same element(s) within a single statement. Example:
$('#myDiv').css('color', 'blue').slideUp().slideDown();
14. How do you hide and show elements in jQuery?
Answer: Use the .hide()
and .show()
methods to hide and show elements, respectively. Example:
$('#myDiv').hide(); // Hides the element
$('#myDiv').show(); // Shows the element
15. What are the animate()
method and its purpose?
Answer: The animate()
method is used to create custom animations by changing CSS properties over time. Example:
$('#myDiv').animate({
left: '+=50px',
opacity: 0.5
}, 1000);
16. How do you stop an animation in jQuery?
Answer: Use the .stop()
method to stop currently running animations on the selected elements. Example:
$('#myDiv').stop();
17. Explain the html()
and text()
methods in jQuery.
Answer: The html()
method gets or sets the HTML content of an element, while the text()
method gets or sets the text content. Example:
$('#myDiv').html('<p>New HTML content</p>'); // Sets HTML
var htmlContent = $('#myDiv').html(); // Gets HTML
$('#myDiv').text('New text content'); // Sets text
var textContent = $('#myDiv').text(); // Gets text
18. What is the data()
method in jQuery?
Answer: The data()
method is used to store and retrieve data associated with DOM elements. Example:
$('#myDiv').data('key', 'value'); // Stores data
var value = $('#myDiv').data('key'); // Retrieves data
19. How do you use the removeClass()
, addClass()
, and toggleClass()
methods?
Answer: These methods manipulate CSS classes on elements. Examples:
$('#myDiv').addClass('newClass'); // Adds a class
$('#myDiv').removeClass('oldClass'); // Removes a class
$('#myDiv').toggleClass('toggleClass'); // Toggles a class
20. What is the difference between find()
and children()
in jQuery?
Answer: The find()
method searches for descendant elements within the selected element, while children()
only searches for immediate children. Example:
$('#parent').find('.descendant'); // Finds all descendants with the class 'descendant'
$('#parent').children('.child'); // Finds all immediate children with the class 'child'
Conclusion
These top 20 jQuery interview questions and answers should help freshers prepare effectively for their interviews. Understanding these concepts will give you a solid foundation in jQuery, making it easier to tackle both basic and advanced interview questions. Good luck with your interview preparation!